목록LeetCode 코딩테스트 (34)
무냐의 개발일지
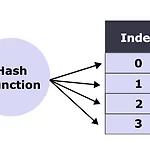
해시테이블은 기본적으로 dictionary라고 생각하면 된다 요런 느낌으로, hash function에 따라 키의 주소가 부여되고, 그 주소에 저장되게 된다특징으로는1. One - waykey를 넣어서 ==> full dict & 주소가 나오는거지그 반대로 주소를 넣어서 key를 얻을수는 없다 2. Deterministic곧, 특정 Hash function에 의해서 특정 주소가 반환된다고 기대할 수 있다는 것.랜덤이 아니라는 말이다. * Collision : 같은 주소에 2개 이상의 값이 틀어갈 때 충돌이 발생하는 것 대표적인 충돌 해결 방법* Chainigng : ex) seperate chaining : 같은 주소에 hashing된 여러개의 dict을 Linked List로 연결해서 집어넣는 방..
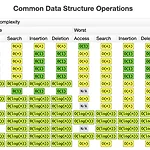
| 기본구조 트리는 기본적으로 루트와 왼쪽, 오른쪽 노드가 있다class Node: def __init__(self, value): self.value = value self.left = None self.right = None class BinarySearchTree: def __init__(self): self.root = None | Insert method def insert(self, value): new_node = Node(value) if self.root == None: self.root = new_node return True cu..
👩💻 문제Objective:The task is to develop a method that takes a sorted list of integers as input and constructs a height-balanced BST.This involves creating a BST where the depth of the two subtrees of any node does not differ by more than one.Achieving a height-balanced tree is crucial for optimizing the efficiency of tree operations, ensuring that the BST remains efficient for search, insertion..
👩💻 문제BST: Invert Binary Tree ( ** Interview Question)Objective: Write a method to invert (or mirror) a binary tree. This means that for every node in the binary tree, you will swap its left and right children. 💡 풀이 def invert(self): self.root = self.__invert_tree(self.root) def __invert_tree(self, current): if current == None: return None ..
| GET (key 값에 해당하는 value를 반환한다) 1. 'apple' 키에 해당하는 값을 반환합니다. 2. 'orange' 키가 존재하지 않기 때문에 None을 반환합니다.3. 'orange' 키가 존재하지 않지만, 기본값으로 'N/A'를 지정해주었기 때문에 'N/A'를 반환합니다. get('key', 0) : key에 해당하는 value를 반환하지만, 그 딕셔너리에 해당 키가 존재하지 않는다면 0으로 지정한다value = my_dict.get('apple')print(value) # 출력: 1value = my_dict.get('orange')print(value) # 출력: Nonevalue = my_dict.get('orange', 'N/A')print(value) # 출력: N/A ..
👩💻 문제You are given a doubly linked list.Implement a method called swap_pairs within the class that swaps the values of adjacent nodes in the linked list. The method should not take any input parameters.Note: This DoublyLinkedList does not have a tail pointer which will make the implementation easier.Example:1 2 3 4 should become 2 1 4 3Your implementation should handle edge cases such a..
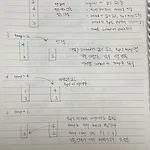
👩💻 문제The sort_stack function takes a single argument, a Stack object. The function should sort the elements in the stack in ascending order (the lowest value will be at the top of the stack) using only one additional stack. The function should use the pop, push, peek, and is_empty methods of the Stack object. 작은 숫자가 맨 위에 오도록 정렬하라 💡 풀이def sort_stack(stack): sorted_stack = Stack() while..
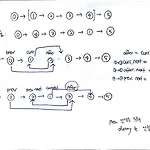
👩💻 문제You are given a singly linked list and two integers start_index and end_index.Your task is to write a method reverse_between within the LinkedList class that reverses the nodes of the linked list from start_index to end_index (inclusive using 0-based indexing) in one pass and in-place.Note: the Linked List does not have a tail which will make the implementation easier.Assumption: You ca..